ease - in - out | ||
---|---|---|
Sinusoidal |
||
![]() ![]() |
.inOutSine()
Description
This function calculates an ease-in-out interpolation which follows a sinusoidal curve, providing a transition between a smooth start and gentle deceleration.
The returned value is by default a nonlinear interpolation between 0.0 and 1.0 or
in case start and stop parameter are being passed as aguments, the output will be an interpolation between those two given values.
The counter
parameter should be tuned according to the totalLength
e.g. if the total totalLength
value was set to 3.0
seconds, the counter
parameter should be an incremental value between 0.0
and 3.0
in order to complete one motion cycle.
There are three kind of cycling behaviour: either "loop"
"alternate"
or "once"
, and when combined with an incremental counter
parameter, the output will variate.
-
"loop"
the motion will loop from0
to1
(this is is the default option);
note: the function expects thecounter
to be between0.0
andtotalLength
-
"alternate"
the motion goes from0
to1
, then inverts from1
back to0
, alternatively.
note: the function expects thecounter
to be between0.0
and twice thetotalLength
-
"once"
the motion cycle from from0
to1
only once
Example:
import easy.ease.*;
float intensity = 4.0;
float totalLength = 2;
float span = 1.5;
float delay = 0.25;
EasyEase curve = new EasyEase(this, intensity, totalLength, span, delay);
void setup() {
size(600, 400);
}
void draw() {
background(#f1f1f1);
fill(#ff0000);
float mot_counter = curve.framer(frameCount);
float x = curve.inOutSine(mot_counter ) * (width-100);
rect(x, 0, 100, width);
}
Ease_inOutSine.pde
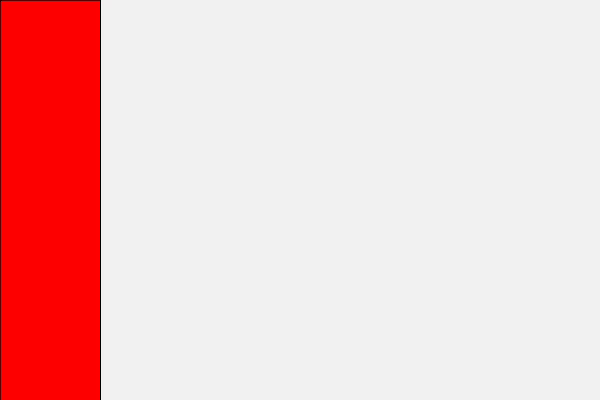
Syntax
.inOutSine(counter)
.inOutSine(counter,option)
.inOutSine(counter, start, stop)
.inOutSine(counter, start, stop, option)
Parameters
counter
(float) an incremental value between 0
and totalLength
start
(float) the lowest desired output value
stop
(float) the highest desired output value
option
(String) either "loop"
, "alternate"
or "once"
Return
float