Constructor Functions
The EasyEase Class can be initialized in different ways, enhancing flexibility and customization
There are 3 different constructor functions each of them initializes an instances of the Class according to the provided arguments
Constructor #1 - default constructor
Constructs an instance of the EasyEase Class expecting only this
, a reference to the current Sketch, as a argument.
It assigns default values for the global parameters like intesity
, totalLength
, curveSpan
, and delay
.
Example:
import easy.ease.*;
//construct an instance of the EaseEase Class called curve
// using "this" as a reference to the current sketch
EasyEase curve = new EasyEase(this);
void setup() {
size(600, 400);
stroke(#ff0000);
noFill();
strokeWeight(3);
loadPixels();
for (float x = 0; x < width; x++) {
for (float y = 0; y < height; y++) {
int index = int(x + y * width);
color c = color(curve.inOutSine(x / width) * 255);
pixels[index] = c;
}
}
updatePixels();
beginShape();
for (float i = 0; i < width; i++) {
vertex(i,(1 - curve.inOutSine((i / width))) * (height - 4) + 2);
}
endShape();
}
constructor_01.pde
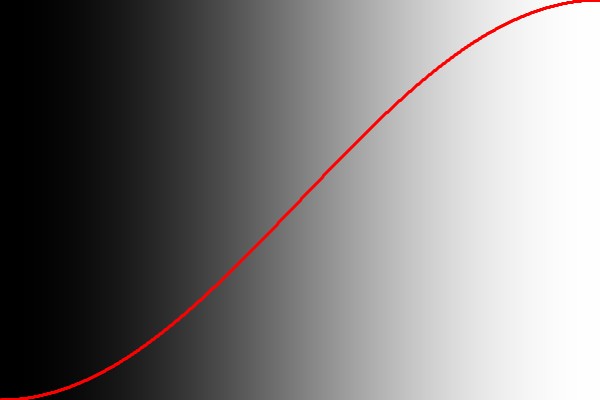
Syntax
Parameters
this
(PApplet) the current sketch
Returns
Object
Constructor #2 - with exponential intensity
Constructs an instance of the EasyEase Class expecting
this
as a reference to the current sketch, and- a
float
value for theintensity
as arguments.
It assigns default values for other global parameters intesity
, totalLength
, curveSpan
, and delay
.
Example:
import easy.ease.*;
// construct an instance of the EaseEase Class called curve
// passing "this" as a reference to the current sketch
// and a float value for the intensity
float intensity = 9.2;
EasyEase curve = new EasyEase(this, intensity);
void setup() {
size(600, 400);
stroke(#ff0000);
noFill();
strokeWeight(3);
loadPixels();
for (float x = 0; x < width; x++) {
for (float y = 0; y < height; y++) {
int index = int(x + y * width);
color c = color(curve.inOut( (x / width))*255);
pixels[index] = c;
}
}
updatePixels();
beginShape();
for (float i = 0; i < width; i++) {
vertex(i, (1-curve.inOut( (i / width)))*(height-4)+2 );
}
endShape();
}
constructor_02.pde
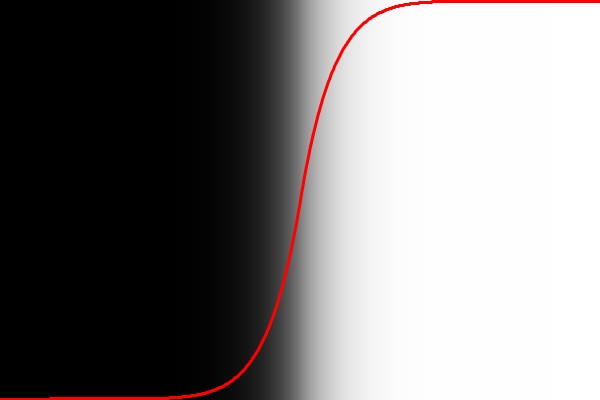
Syntax
Parameters
this
(PApplet) the current sketch
intensity
(float) The intensity / exponential value of the easing curve.
Returns
Object
Constructor #3 - with exponential intensity and time related parameters
Constructs an instance of the EasyEase Class expecting
this
as a reference to the current sketch,- a
float
value for theintensity
, - a
float
value for thetotalLength
, - a
float
value for thecurveSpan
, - a
float
value for thedelay
as arguments.
Example:
import easy.ease.*;
// construct an instance of the EaseEase Class called curve
// passing "this" as a reference to the current sketch
// and float values for the intensity, totalLength,
// curveSpan and delay
float intensity = 4.3;
float totalLength = 1;
float curveSpan = 0.4;
float delay = 0.6;
EasyEase curve = new EasyEase(this, intensity, totalLength, curveSpan, delay);
void setup() {
size(600, 400);
stroke(#ff0000);
noFill();
strokeWeight(3);
loadPixels();
for (float x = 0; x < width; x++) {
for (float y = 0; y < height; y++) {
int index = int(x + y * width);
color c = color(curve.out( (x / width))*255);
pixels[index] = c;
}
}
updatePixels();
beginShape();
for (float i = 0; i < width; i++) {
vertex(i, (1-curve.out( (i / width)))*(height-4)+2 );
}
endShape();
}
constructor_03.pde
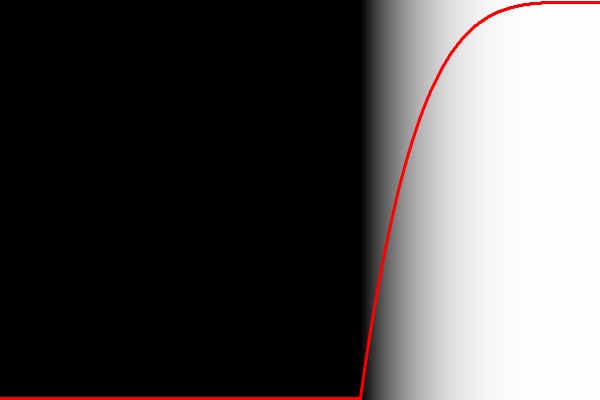
Syntax
Parameters
this
(PApplet) the current sketch
intensity
(float) The intensity / exponential value of the easing curve.
totalLength
(float) The total length (in seconds) of the interpolation / motion
curveSpan
(float) The span (in seconds) of the easing curve.
delay
(float) the delay (in seconds) before the curve starts bending
Returns
Object